Authentication: Register & Login with JWT in PHP & MYSQL
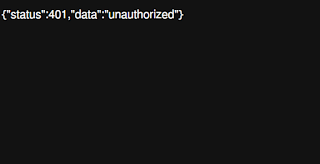
In the previous tutorial, you learnt to upload form data containing text fields and and image . This tutorial teaches you how to do authentication in PHP & MYSQL using JWT and restrict APIs to allow only authenticated users. From a client app, a visitor can register a new user account using username, email, and password. The password is encrypted using PHP password_hash function before saving in database. The registered user logs in to the system using username and password. Behind the scene, after a successful login, a valid token will be generated on the server and sent to the client. Then whenever the client accesses the restricted APIs, it must send the token to be verified on the server. To generate and verify token in PHP, in the api folder, run the following command to install php-jwt: api>composer require firebase/php-jwt Execute the following command to create a migration file to create jwtusers. ./vendor/bin/phinx create JwtUsersTableMigration <?php declare (s